TL, DR
PySimpleGUI is one of the easiest way to create GUIs (Graphical User Interfaces) for your Python applications. If you use PySimpleGUI you should consider supporting the project with their official Udemy course here.
Python and GUIs
In most of the cases, when we develop Python applications or scripts we mostly operate via command line or in Jupyter notebooks. However, if we want to distribute our application to more users those are not the most attractive ways to do it.
There are several Python packages that help you creating GUIs for your applications. Among them, PySimpleGUI is the easiest to implement also for non-experienced programmers. You can install PySimpleGUI with pip as follow:
pip install pysimplegui
PySimpleGUI act as a wrapper on top of other GUI libraries – like tkinter, Qt, WxPython, and Remi – doing all the heavy lifting and making them very simple to use.
A simple example
In this post I will show a very simple application that grab a kitten picture from placekitten APIs and displays it together with current date and time information. We will use a few other Python libraries like Requests, Pillows, and Datetime to handle all functionalities.
I am using the Qt port of PySimpleGUI, as on my old Macbook I have an outdated Tkinter version that I cannot update for other dependencies. First thing, let’s import all libraries we are going to use:
import PySimpleGUIQt as sg
import requests
from PIL import Image
import io
from datetime import datetime
Requests will be used to get the cat picture from placekitten API. PIL and io will help us to handle the image and prepare it for display. Datetime will give us the current date and time for our clock.
Then we move on to retrieve our cute kitten image and save it in memory. We also create the initial time and date string for the clock.
cat_bytes = requests.get("https://placekitten.com/200/300")
cat_image = Image.open(io.BytesIO(cat_bytes.content))
cat_png = io.BytesIO()
cat_image.save(cat_png, format="PNG")
cat_display = cat_png.getvalue()
time_text = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
Now it’s time to create the layout and window for our application. We will also include an “Exit” button to close it. All it’s done in a few lines.
layout = [
[sg.Text(time_text, key="time", font="Arial 24")],
[sg.Image(data=cat_display)],
[sg.Button('Exit')]
]
window = sg.Window("Clock with cat image", layout)
Finally we create the event loop that will display our window and update the time. The last line will close the windows when we exit our program.
while True:
event, values = window.read(timeout=10)
if event == sg.WIN_CLOSED or event == 'Exit':
break
new_time_text = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
window["time"].update(new_time_text)
window.close()
Here you can see the full script. In about 28 lines of code, including blank lines and indentations for better readability, our self-updating GUI application is done!
import PySimpleGUIQt as sg
import requests
from PIL import Image
import io
from datetime import datetime
cat_bytes = requests.get("https://placekitten.com/200/300")
cat_image = Image.open(io.BytesIO(cat_bytes.content))
cat_png = io.BytesIO()
cat_image.save(cat_png, format="PNG")
cat_display = cat_png.getvalue()
time_text = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
layout = [
[sg.Text(time_text, key="time", font="Arial 24")],
[sg.Image(data=cat_display)],
[sg.Button('Exit')]
]
window = sg.Window("Clock with cat image", layout)
while True:
event, values = window.read(timeout=10)
if event == sg.WIN_CLOSED or event == 'Exit':
break
new_time_text = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
window["time"].update(new_time_text)
window.close()
This is the result, as you can see yourself if you run the script:
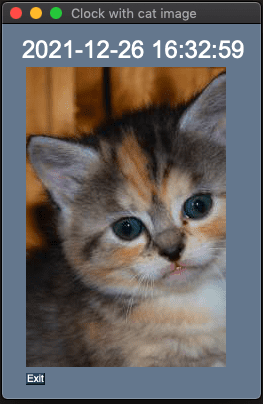
You can find this and more example in this Github repository.
PySimpleGUI summary
To sum everything up, if you want to move your Python applications to the next level and provide GUIs for your uses, you should try PySimpleGUI. The project needs financial support, so if you use it you should consider their official Udemy course here.
Related links
- PySimpleGUI official Udemy course link
- PySimpleGUI documentation link
- placekitten APIs link
- Github repository with examples link
Do you like our content? Check more of our posts in our blog!